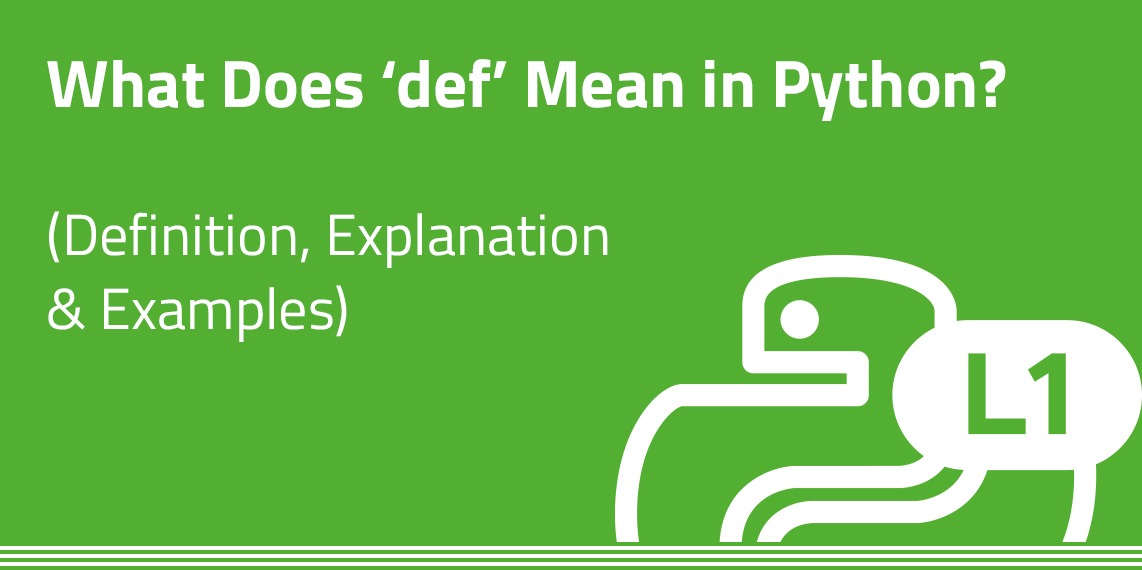
What Does ‘def’ Mean in Python? (Definition, Explanation & Examples)
Python is a popular programming language that kids as young as 11 years old can learn. In the working world, it is widely used in applications ranging from web development to scientific computing. But at FunTech, kids can learn how to make games using it.
During our Python courses, ‘def’ is one of the most important concepts in Python that will be taught. But just what is ‘def’ in Python, and how should it be used?
In Python, ‘def’ stands for definition and is used to create or define a function. When a programmer wants to create a new function, they use the def keyword followed by the function name. The function name should be descriptive and should convey the purpose of the function.
But how do you use ‘def’ in Python? Here’s are some examples in an easy way that kids can understand.
7 examples of how to use ‘def’ in Python
1. Hello, World! Function
This function prints “Hello, World!” when called.
def say_hello():
print("Hello, World!")
# Calling the function
say_hello()
2. Adding Two Numbers
This function takes two numbers as input and returns their sum.
def add_numbers(a, b):
return a + b
# Calling the function
result = add_numbers(3, 4)
print("The sum is:", result)
3. Greeting a Person
This function takes a person’s name as input and prints a personalised greeting.
def greet(name):
print(f"Hello, {name}!")
# Calling the function
greet("Alice")
4. Finding the Largest Number
This function takes three numbers and returns the largest one.
def find_largest(a, b, c):
if a > b and a > c:
return a
elif b > a and b > c:
return b
else:
return c
# Calling the function
largest = find_largest(3, 7, 5)
print("The largest number is:", largest)
5. Checking if a Number is Even
This function checks if a given number is even and returns True or False.
def is_even(number):
return number % 2 == 0
# Calling the function
print("Is 4 even?", is_even(4))
print("Is 7 even?", is_even(7))
6. Counting Down
This function counts down from a given number to zero.
def countdown(n):
while n >= 0:
print(n)
n -= 1
# Calling the function
countdown(5)
7. Drawing a Square (using Turtle graphics)
This function draws a square using the Turtle graphics library.
import turtle
def draw_square():
for _ in range(4):
turtle.forward(100)
turtle.right(90)
# Calling the function
turtle.speed(1)
draw_square()
turtle.done()
Kids can learn Python with FunTech
Keep me Informed
Be the first to know about Flash and Early Bird Sales as well as new courses, summer locations and more.
KEEP ME INFORMED